Projects
On this page are a few of my personal projects which I am both particularly proud of as well as think other people may find of interest. With that said, other projects of mine in addition to this found here can be located on my Github.
Programming Languages
Owlscript
Owlscript is my dynamically typed and lexically scoped, garbage collected scripting language developed with support for procedural and functional paradigms in mind. Some noteable features include dynamic object creation via struct types, an integrated list type with a plethora of supporting operations including map/sort/filter, as well as first class functions with lambda expressions and closures. Owlscript also sports first class strings with support for concatenation, interpolation, and regular expression matching.
This rich combination of features combine to result in a language that is both at once familiar, and also fresh and new. The following code samples highlight some of the features just mentioned.
def fib(var n) {
if (n < 2) {
return 1;
} else {
return fib(n-1)+fib(n-2);
}
}
def main() {
let fibs := map([5,6,7,8,9], fib);
println fibs;
println filter(fibs, &(i) -> i % 2 == 0);
println first(fibs);
println rest(fibs);
}
max@thinkpad:~$ owlscript -f fib.owl
[ 8, 13, 21, 34, 55 ]
[ 8, 34]
8
[ 13, 21, 34, 55 ]
max@thinkpad:~$
In addition to running scripts from files, Owlscript features an integrated read-eval-print loop (REPL) for interactive development sessions:
max@thinkpad:~/owlscript$ owlscript
Owlscript(0)> let multiplyAndIncrement := &(i) -> 3*i+1;
Owlscript(1)> let count := 1;
Owlscript(2)> while (count < 10) { println multiplyAndIncrement(count); count := count + 1; }
4
7
10
13
16
19
22
25
28
Owlscript(3)>
Owlscript is in continuous development and is constantly evolving. Its main purpose is to facilitate my personal exploration of compiler/interpreter implementation and programming language design. I am currently in the process of extending the tree walking interpreter to include a pcode compiler, though this is still in the early phases of development.
mgcLisp
What language geek hasn't implemented a lisp interpreter? After a long weekend spent at a friends lake house reading through The Structure And Interpretation of Computer Programs I decided it was time to give it another whack with this being the result of that effort. Anyone interested in implementing a lisp interpreter in C/C++ or similar language may find it of interest.
max@MaxGorenLaptop:/mnt/c/Users/mgoren/Documents/GitHub/rlisp$ ./mgclisp
[mgclisp repl]
mgclisp(1)> (define fib (lambda (x) (if (< x 2) 1 (+ (fib (- x 1)) (fib (- x 2))))))
fib
mgclisp(2)> (fib 6)
13
mgclisp(3)> quit
max@MaxGorenLaptop:/mnt/c/Users/mgoren/Documents/GitHub/rlisp$
Utilities
mgczip
Lossless data compression! mgczip supports compression using either huffman coding or LZW to compress and uncompress files. Depending on the algorithm chosen and the file being compressed, many files can be reduced in size by 40 - 50%.
max@MaxGorenLaptop:/mnt/c/Users/mgoren/Documents/GitHub/ThompsonsConstruction$ mgczip -cl parser.hpp
Starting size: 6260 bytes
Ending size: 2994 bytes
0.521725 compression ratio.
max@MaxGorenLaptop:/mnt/c/Users/mgoren/Documents/GitHub/ThompsonsConstruction$ mgczip -ch parser.hpp
Starting size: 6260 bytes
Ending size: 3349 bytes
0.465016 compression ratio.
max@MaxGorenLaptop:/mnt/c/Users/mgoren/Documents/GitHub/ThompsonsConstruction$
In the above example, I compress the parser header for my regular expression engine. Using the LZW algorithm to compress the file results in 52% reduction in space. Huffman coding is then used on the same file and reaches a slightly less but still respectable 46% compression ratio.
CodeBlahger
CodeBlahger is my personal blogging platform/content management system and the application used to serve the site you are currently viewing! Not satisfied with the PHP based ecosystems of Drupal and Wordpress, not to mention the seemingly never ending security updates that accompanies a self-hosted instance of one, I decided to take matters into my own hands and created CodeBlahger.
I wanted to use a similar tech stack to what I use professionally as backend software engineer and so decided to develop CodeBlahger in Java using the Springboot framework and MySQL for the backend. The front end is rendered using the thymeleaf templating library and HTML5, CSS, and some light javascript. Being a backend developer by trade, creating CodeBlahgers layout took me far longer than id like to admit, but I'm happy with the result.
CodeBlahger features an integrated WYSIWYG editor for creating and editing blogposts, syntax highlighting on code examples, and comment moderation. Additionally, posts can be browsed by category as well as featuring full text search on all posts. Thanks to containerization via docker, deployment is a breeze. Once deployed, CodeBlahger requires remarkably few resources, able to run comfortably on a relatively low-spec VPS.
Data Structure & Algorithm Visualization
VizSort
Visualize the way different sorting algorithms move data during sorting to gain a deeper understanding of how they work! Developed in C++, VizSort depends on the SFML library for graphics rendering. With a wide selection of algorithms to choose from, its easy to become immersed in following the rearrangemnet of data, as if "peeling open" the algorithm to visually inspect how each algorithm works.
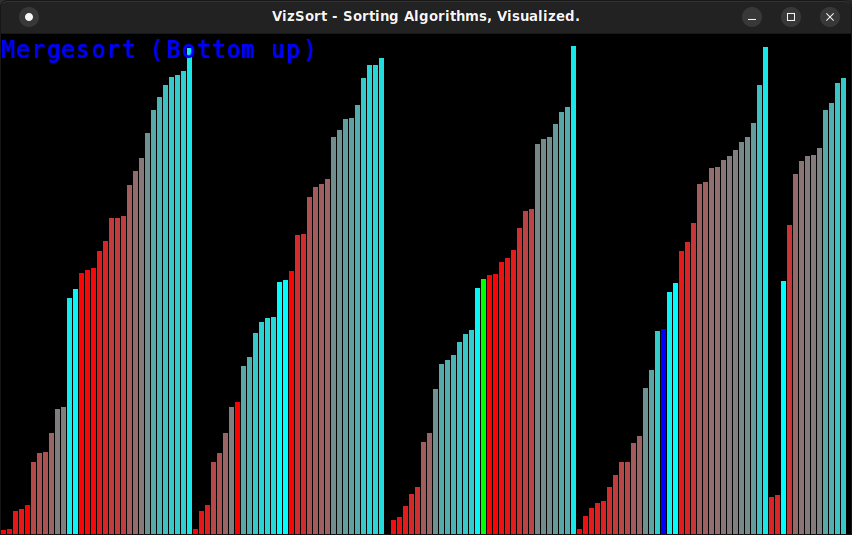
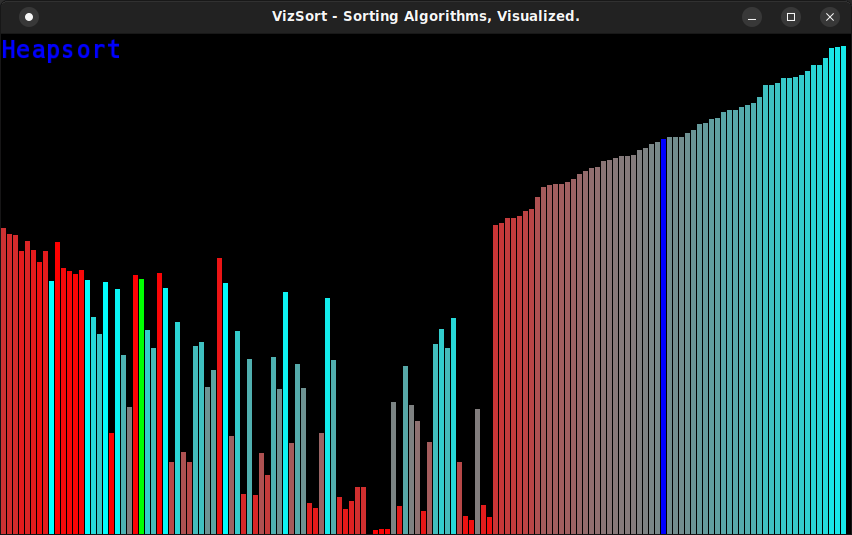
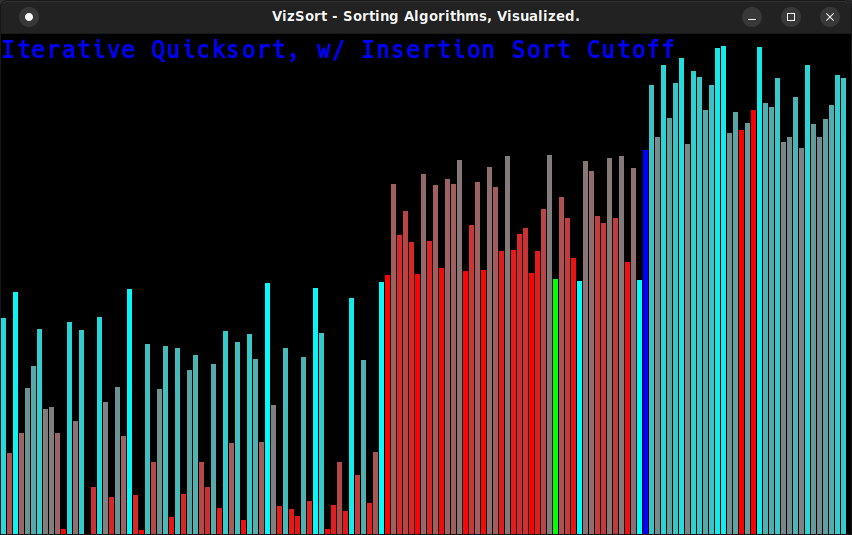
Binary Tree Visualizer
This is a simple tool developed in C++ using SFML to generate the images of the various types of binary search trees that I discuss in my posts. It was particularly useful when I decided to deep dive the various types of Red Black trees.
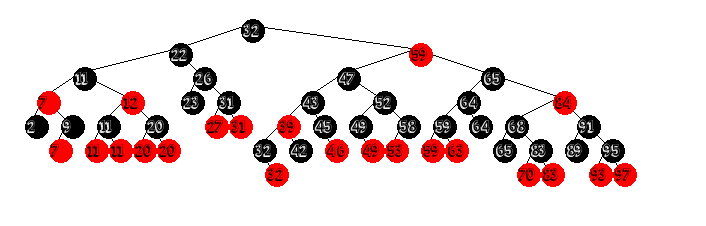
-
Lossless Compression Part III: Huffman Coding
-
Lossless Compression Part II: The LZ77 Algorithm
-
Lossless Compression Part I: Working with Bits in a Byte Oriented World
-
Bottom Up AVL Tree: The OG Self-Balancing Binary Search Tree
-
A Different Take on Merge Sort: Binary Search Trees?
-
Deleting Entries From Open-Address Hash tables
-
Transform any Binary Search Tree in to a Sorted Linked List
-
From Regular Expressions To NFA by way of Abstract Syntax Trees: Thompsons Construction
-
Extending Sedgewick's explicit Digraph NFA
-
Iterative In-order B Tree Traversal